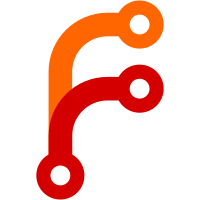
When building kernel without CONFIG_FRAME_POINTER, gcc uses CFA (canonical frame address) for frame base. With this patch, perf probe just gets CFI (call frame information) from debuginfo and search corresponding CFA from the CFI. IOW, this allows perf probe works correctly on the kernel without CONFIG_FRAME_POINTER. <Before> ./perf probe -fn sched_slice:12 lw.weight Fatal: DW_OP 156 is not supported. (^^^ DW_OP_call_frame_cfa) <After> ./perf probe -fn sched_slice:12 lw.weight Add new event: probe:sched_slice (on sched_slice:12 with weight=lw.weight) Cc: Ingo Molnar <mingo@elte.hu> Cc: Paul Mackerras <paulus@samba.org> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Mike Galbraith <efault@gmx.de> Cc: Frederic Weisbecker <fweisbec@gmail.com> LKML-Reference: <20100412171728.3790.98217.stgit@localhost6.localdomain6> Signed-off-by: Masami Hiramatsu <mhiramat@redhat.com> Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com>
63 lines
1.7 KiB
C
63 lines
1.7 KiB
C
#ifndef _PROBE_FINDER_H
|
|
#define _PROBE_FINDER_H
|
|
|
|
#include <stdbool.h>
|
|
#include "util.h"
|
|
#include "probe-event.h"
|
|
|
|
#define MAX_PATH_LEN 256
|
|
#define MAX_PROBE_BUFFER 1024
|
|
#define MAX_PROBES 128
|
|
|
|
static inline int is_c_varname(const char *name)
|
|
{
|
|
/* TODO */
|
|
return isalpha(name[0]) || name[0] == '_';
|
|
}
|
|
|
|
#ifdef DWARF_SUPPORT
|
|
/* Find kprobe_trace_events specified by perf_probe_event from debuginfo */
|
|
extern int find_kprobe_trace_events(int fd, struct perf_probe_event *pev,
|
|
struct kprobe_trace_event **tevs);
|
|
|
|
/* Find a perf_probe_point from debuginfo */
|
|
extern int find_perf_probe_point(int fd, unsigned long addr,
|
|
struct perf_probe_point *ppt);
|
|
|
|
extern int find_line_range(int fd, struct line_range *lr);
|
|
|
|
#include <dwarf.h>
|
|
#include <libdw.h>
|
|
|
|
struct probe_finder {
|
|
struct perf_probe_event *pev; /* Target probe event */
|
|
struct kprobe_trace_event *tevs; /* Result trace events */
|
|
int ntevs; /* number of trace events */
|
|
|
|
/* For function searching */
|
|
int lno; /* Line number */
|
|
Dwarf_Addr addr; /* Address */
|
|
const char *fname; /* Real file name */
|
|
Dwarf_Die cu_die; /* Current CU */
|
|
struct list_head lcache; /* Line cache for lazy match */
|
|
|
|
/* For variable searching */
|
|
Dwarf_CFI *cfi; /* Call Frame Information */
|
|
Dwarf_Op *fb_ops; /* Frame base attribute */
|
|
struct perf_probe_arg *pvar; /* Current target variable */
|
|
struct kprobe_trace_arg *tvar; /* Current result variable */
|
|
};
|
|
|
|
struct line_finder {
|
|
struct line_range *lr; /* Target line range */
|
|
|
|
const char *fname; /* File name */
|
|
int lno_s; /* Start line number */
|
|
int lno_e; /* End line number */
|
|
Dwarf_Die cu_die; /* Current CU */
|
|
int found;
|
|
};
|
|
|
|
#endif /* DWARF_SUPPORT */
|
|
|
|
#endif /*_PROBE_FINDER_H */
|