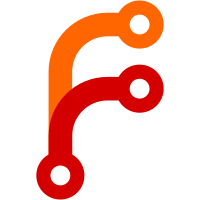
Otherwise lock debugging messages on runqueue locks can deadlock the system due to the wakeups performed by printk(). Signed-off-by: David S. Miller <davem@davemloft.net> Signed-off-by: Ingo Molnar <mingo@elte.hu>
47 lines
1.1 KiB
C
47 lines
1.1 KiB
C
/*
|
|
* lib/debug_locks.c
|
|
*
|
|
* Generic place for common debugging facilities for various locks:
|
|
* spinlocks, rwlocks, mutexes and rwsems.
|
|
*
|
|
* Started by Ingo Molnar:
|
|
*
|
|
* Copyright (C) 2006 Red Hat, Inc., Ingo Molnar <mingo@redhat.com>
|
|
*/
|
|
#include <linux/kernel.h>
|
|
#include <linux/rwsem.h>
|
|
#include <linux/mutex.h>
|
|
#include <linux/module.h>
|
|
#include <linux/spinlock.h>
|
|
#include <linux/debug_locks.h>
|
|
|
|
/*
|
|
* We want to turn all lock-debugging facilities on/off at once,
|
|
* via a global flag. The reason is that once a single bug has been
|
|
* detected and reported, there might be cascade of followup bugs
|
|
* that would just muddy the log. So we report the first one and
|
|
* shut up after that.
|
|
*/
|
|
int debug_locks = 1;
|
|
|
|
/*
|
|
* The locking-testsuite uses <debug_locks_silent> to get a
|
|
* 'silent failure': nothing is printed to the console when
|
|
* a locking bug is detected.
|
|
*/
|
|
int debug_locks_silent;
|
|
|
|
/*
|
|
* Generic 'turn off all lock debugging' function:
|
|
*/
|
|
int debug_locks_off(void)
|
|
{
|
|
if (xchg(&debug_locks, 0)) {
|
|
if (!debug_locks_silent) {
|
|
oops_in_progress = 1;
|
|
console_verbose();
|
|
return 1;
|
|
}
|
|
}
|
|
return 0;
|
|
}
|