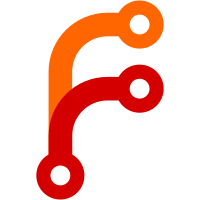
In a few places in the kernel, the code prints a human-readable USB device speed (eg. "high speed"). This involves a switch statement sometimes wrapped around in ({ ... }) block leading to code repetition. To mitigate this issue, this commit introduces usb_speed_string() function, which returns a human-readable name of provided speed. It also changes a few places switch was used to use this new function. This changes a bit the way the speed is printed in few instances at the same time standardising it. Signed-off-by: Michal Nazarewicz <mina86@mina86.com> Signed-off-by: Greg Kroah-Hartman <gregkh@suse.de>
35 lines
1.1 KiB
C
35 lines
1.1 KiB
C
/*
|
|
* Provides code common for host and device side USB.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation, version 2.
|
|
*
|
|
* If either host side (ie. CONFIG_USB=y) or device side USB stack
|
|
* (ie. CONFIG_USB_GADGET=y) is compiled in the kernel, this module is
|
|
* compiled-in as well. Otherwise, if either of the two stacks is
|
|
* compiled as module, this file is compiled as module as well.
|
|
*/
|
|
|
|
#include <linux/kernel.h>
|
|
#include <linux/module.h>
|
|
#include <linux/usb/ch9.h>
|
|
|
|
const char *usb_speed_string(enum usb_device_speed speed)
|
|
{
|
|
static const char *const names[] = {
|
|
[USB_SPEED_UNKNOWN] = "UNKNOWN",
|
|
[USB_SPEED_LOW] = "low-speed",
|
|
[USB_SPEED_FULL] = "full-speed",
|
|
[USB_SPEED_HIGH] = "high-speed",
|
|
[USB_SPEED_WIRELESS] = "wireless",
|
|
[USB_SPEED_SUPER] = "super-speed",
|
|
};
|
|
|
|
if (speed < 0 || speed >= ARRAY_SIZE(names))
|
|
speed = USB_SPEED_UNKNOWN;
|
|
return names[speed];
|
|
}
|
|
EXPORT_SYMBOL_GPL(usb_speed_string);
|
|
|
|
MODULE_LICENSE("GPL");
|