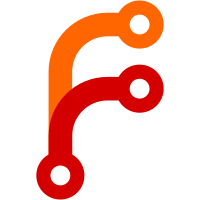
MSMs post 8x50 have 2Mb at the beginning of RAM reserved for shared memory. Since the kernel hasn't typically been told this RAM exists, PHYS_OFFSET has been set to 0xN0200000 and the memory atags passed to the kernel have matched. This doesn't play nicely with things such as AUTO_ZRELADDR, which doesn't work at all, and dynamic phys to virt, which requires an MSM specific workaround. Work around these issues by telling the kernel RAM starts at 0xN0000000 (it actually does) and fixup the atags from the bootloader (if necessary) to say the same. In addition, make sure to set TEXT_OFFSET at least 2Mb beyond the start of RAM so that the kernel doesn't end up being decompressed into shared memory. After doing this, AUTO_ZRELADDR should work on MSM with no problems and ARM_PATCH_PHYS_VIRT_16BIT should no longer be necessary. Signed-off-by: Stephen Boyd <sboyd@codeaurora.org> Acked-by: Nicolas Pitre <nicolas.pitre@linaro.org> Acked-by: David Brown <davidb@codeaurora.org> Signed-off-by: Russell King <rmk+kernel@arm.linux.org.uk>
152 lines
4 KiB
C
152 lines
4 KiB
C
/* Copyright (c) 2009-2010, Code Aurora Forum. All rights reserved.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 and
|
|
* only version 2 as published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
|
|
* 02110-1301, USA.
|
|
*/
|
|
|
|
#include <linux/kernel.h>
|
|
#include <linux/irq.h>
|
|
#include <linux/gpio.h>
|
|
#include <linux/platform_device.h>
|
|
#include <linux/delay.h>
|
|
#include <linux/io.h>
|
|
#include <linux/smsc911x.h>
|
|
#include <linux/usb/msm_hsusb.h>
|
|
#include <linux/clkdev.h>
|
|
#include <linux/memblock.h>
|
|
|
|
#include <asm/mach-types.h>
|
|
#include <asm/mach/arch.h>
|
|
#include <asm/memory.h>
|
|
#include <asm/setup.h>
|
|
|
|
#include <mach/gpio.h>
|
|
#include <mach/board.h>
|
|
#include <mach/msm_iomap.h>
|
|
#include <mach/dma.h>
|
|
|
|
#include <mach/vreg.h>
|
|
#include "devices.h"
|
|
#include "gpiomux.h"
|
|
#include "proc_comm.h"
|
|
|
|
extern struct sys_timer msm_timer;
|
|
|
|
static void __init msm7x30_fixup(struct machine_desc *desc, struct tag *tag,
|
|
char **cmdline, struct meminfo *mi)
|
|
{
|
|
for (; tag->hdr.size; tag = tag_next(tag))
|
|
if (tag->hdr.tag == ATAG_MEM && tag->u.mem.start == 0x200000) {
|
|
tag->u.mem.start = 0;
|
|
tag->u.mem.size += SZ_2M;
|
|
}
|
|
}
|
|
|
|
static void __init msm7x30_reserve(void)
|
|
{
|
|
memblock_remove(0x0, SZ_2M);
|
|
}
|
|
|
|
static int hsusb_phy_init_seq[] = {
|
|
0x30, 0x32, /* Enable and set Pre-Emphasis Depth to 20% */
|
|
0x02, 0x36, /* Disable CDR Auto Reset feature */
|
|
-1
|
|
};
|
|
|
|
static struct msm_otg_platform_data msm_otg_pdata = {
|
|
.phy_init_seq = hsusb_phy_init_seq,
|
|
.mode = USB_PERIPHERAL,
|
|
.otg_control = OTG_PHY_CONTROL,
|
|
};
|
|
|
|
struct msm_gpiomux_config msm_gpiomux_configs[GPIOMUX_NGPIOS] = {
|
|
#ifdef CONFIG_SERIAL_MSM_CONSOLE
|
|
[49] = { /* UART2 RFR */
|
|
.suspended = GPIOMUX_DRV_2MA | GPIOMUX_PULL_DOWN |
|
|
GPIOMUX_FUNC_2 | GPIOMUX_VALID,
|
|
},
|
|
[50] = { /* UART2 CTS */
|
|
.suspended = GPIOMUX_DRV_2MA | GPIOMUX_PULL_DOWN |
|
|
GPIOMUX_FUNC_2 | GPIOMUX_VALID,
|
|
},
|
|
[51] = { /* UART2 RX */
|
|
.suspended = GPIOMUX_DRV_2MA | GPIOMUX_PULL_DOWN |
|
|
GPIOMUX_FUNC_2 | GPIOMUX_VALID,
|
|
},
|
|
[52] = { /* UART2 TX */
|
|
.suspended = GPIOMUX_DRV_2MA | GPIOMUX_PULL_DOWN |
|
|
GPIOMUX_FUNC_2 | GPIOMUX_VALID,
|
|
},
|
|
#endif
|
|
};
|
|
|
|
static struct platform_device *devices[] __initdata = {
|
|
#if defined(CONFIG_SERIAL_MSM) || defined(CONFIG_MSM_SERIAL_DEBUGGER)
|
|
&msm_device_uart2,
|
|
#endif
|
|
&msm_device_smd,
|
|
&msm_device_otg,
|
|
&msm_device_hsusb,
|
|
&msm_device_hsusb_host,
|
|
};
|
|
|
|
static void __init msm7x30_init_irq(void)
|
|
{
|
|
msm_init_irq();
|
|
}
|
|
|
|
static void __init msm7x30_init(void)
|
|
{
|
|
msm_device_otg.dev.platform_data = &msm_otg_pdata;
|
|
msm_device_hsusb.dev.parent = &msm_device_otg.dev;
|
|
msm_device_hsusb_host.dev.parent = &msm_device_otg.dev;
|
|
|
|
platform_add_devices(devices, ARRAY_SIZE(devices));
|
|
}
|
|
|
|
static void __init msm7x30_map_io(void)
|
|
{
|
|
msm_map_msm7x30_io();
|
|
msm_clock_init(msm_clocks_7x30, msm_num_clocks_7x30);
|
|
}
|
|
|
|
MACHINE_START(MSM7X30_SURF, "QCT MSM7X30 SURF")
|
|
.boot_params = PLAT_PHYS_OFFSET + 0x100,
|
|
.fixup = msm7x30_fixup,
|
|
.reserve = msm7x30_reserve,
|
|
.map_io = msm7x30_map_io,
|
|
.init_irq = msm7x30_init_irq,
|
|
.init_machine = msm7x30_init,
|
|
.timer = &msm_timer,
|
|
MACHINE_END
|
|
|
|
MACHINE_START(MSM7X30_FFA, "QCT MSM7X30 FFA")
|
|
.boot_params = PLAT_PHYS_OFFSET + 0x100,
|
|
.fixup = msm7x30_fixup,
|
|
.reserve = msm7x30_reserve,
|
|
.map_io = msm7x30_map_io,
|
|
.init_irq = msm7x30_init_irq,
|
|
.init_machine = msm7x30_init,
|
|
.timer = &msm_timer,
|
|
MACHINE_END
|
|
|
|
MACHINE_START(MSM7X30_FLUID, "QCT MSM7X30 FLUID")
|
|
.boot_params = PLAT_PHYS_OFFSET + 0x100,
|
|
.fixup = msm7x30_fixup,
|
|
.reserve = msm7x30_reserve,
|
|
.map_io = msm7x30_map_io,
|
|
.init_irq = msm7x30_init_irq,
|
|
.init_machine = msm7x30_init,
|
|
.timer = &msm_timer,
|
|
MACHINE_END
|