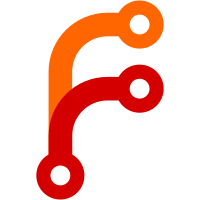
Rename mach-imx/include/mach/common.h to mach-imx/common.h and update all users to include common.h rather than mach/common.h. It also removes an unneeded inclusion to common.h in mach-imx/devices/devices.c. Signed-off-by: Shawn Guo <shawn.guo@linaro.org> Acked-by: Sascha Hauer <s.hauer@pengutronix.de> Acked-by: Arnd Bergmann <arnd@arndb.de>
54 lines
1.1 KiB
C
54 lines
1.1 KiB
C
/*
|
|
* Copyright 2011 Freescale Semiconductor, Inc.
|
|
* Copyright 2011 Linaro Ltd.
|
|
*
|
|
* The code contained herein is licensed under the GNU General Public
|
|
* License. You may obtain a copy of the GNU General Public License
|
|
* Version 2 or later at the following locations:
|
|
*
|
|
* http://www.opensource.org/licenses/gpl-license.html
|
|
* http://www.gnu.org/copyleft/gpl.html
|
|
*/
|
|
|
|
#include <linux/errno.h>
|
|
#include <asm/cacheflush.h>
|
|
#include <asm/cp15.h>
|
|
|
|
#include "common.h"
|
|
|
|
static inline void cpu_enter_lowpower(void)
|
|
{
|
|
unsigned int v;
|
|
|
|
flush_cache_all();
|
|
asm volatile(
|
|
"mcr p15, 0, %1, c7, c5, 0\n"
|
|
" mcr p15, 0, %1, c7, c10, 4\n"
|
|
/*
|
|
* Turn off coherency
|
|
*/
|
|
" mrc p15, 0, %0, c1, c0, 1\n"
|
|
" bic %0, %0, %3\n"
|
|
" mcr p15, 0, %0, c1, c0, 1\n"
|
|
" mrc p15, 0, %0, c1, c0, 0\n"
|
|
" bic %0, %0, %2\n"
|
|
" mcr p15, 0, %0, c1, c0, 0\n"
|
|
: "=&r" (v)
|
|
: "r" (0), "Ir" (CR_C), "Ir" (0x40)
|
|
: "cc");
|
|
}
|
|
|
|
/*
|
|
* platform-specific code to shutdown a CPU
|
|
*
|
|
* Called with IRQs disabled
|
|
*/
|
|
void imx_cpu_die(unsigned int cpu)
|
|
{
|
|
cpu_enter_lowpower();
|
|
imx_enable_cpu(cpu, false);
|
|
|
|
/* spin here until hardware takes it down */
|
|
while (1)
|
|
;
|
|
}
|