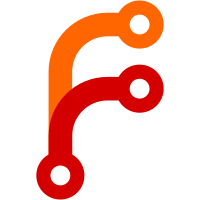
The SO_ATTACH_FILTER option is set only. I propose to add the get ability by using SO_ATTACH_FILTER in getsockopt. To be less irritating to eyes the SO_GET_FILTER alias to it is declared. This ability is required by checkpoint-restore project to be able to save full state of a socket. There are two issues with getting filter back. First, kernel modifies the sock_filter->code on filter load, thus in order to return the filter element back to user we have to decode it into user-visible constants. Fortunately the modification in question is interconvertible. Second, the BPF_S_ALU_DIV_K code modifies the command argument k to speed up the run-time division by doing kernel_k = reciprocal(user_k). Bad news is that different user_k may result in same kernel_k, so we can't get the original user_k back. Good news is that we don't have to do it. What we need to is calculate a user2_k so, that reciprocal(user2_k) == reciprocal(user_k) == kernel_k i.e. if it's re-loaded back the compiled again value will be exactly the same as it was. That said, the user2_k can be calculated like this user2_k = reciprocal(kernel_k) with an exception, that if kernel_k == 0, then user2_k == 1. The optlen argument is treated like this -- when zero, kernel returns the amount of sock_fprog elements in filter, otherwise it should be large enough for the sock_fprog array. changes since v1: * Declared SO_GET_FILTER in all arch headers * Added decode of vlan-tag codes Signed-off-by: Pavel Emelyanov <xemul@parallels.com> Signed-off-by: David S. Miller <davem@davemloft.net>
76 lines
1.7 KiB
C
76 lines
1.7 KiB
C
#ifndef __ASM_GENERIC_SOCKET_H
|
|
#define __ASM_GENERIC_SOCKET_H
|
|
|
|
#include <asm/sockios.h>
|
|
|
|
/* For setsockopt(2) */
|
|
#define SOL_SOCKET 1
|
|
|
|
#define SO_DEBUG 1
|
|
#define SO_REUSEADDR 2
|
|
#define SO_TYPE 3
|
|
#define SO_ERROR 4
|
|
#define SO_DONTROUTE 5
|
|
#define SO_BROADCAST 6
|
|
#define SO_SNDBUF 7
|
|
#define SO_RCVBUF 8
|
|
#define SO_SNDBUFFORCE 32
|
|
#define SO_RCVBUFFORCE 33
|
|
#define SO_KEEPALIVE 9
|
|
#define SO_OOBINLINE 10
|
|
#define SO_NO_CHECK 11
|
|
#define SO_PRIORITY 12
|
|
#define SO_LINGER 13
|
|
#define SO_BSDCOMPAT 14
|
|
/* To add :#define SO_REUSEPORT 15 */
|
|
|
|
#ifndef SO_PASSCRED /* powerpc only differs in these */
|
|
#define SO_PASSCRED 16
|
|
#define SO_PEERCRED 17
|
|
#define SO_RCVLOWAT 18
|
|
#define SO_SNDLOWAT 19
|
|
#define SO_RCVTIMEO 20
|
|
#define SO_SNDTIMEO 21
|
|
#endif
|
|
|
|
/* Security levels - as per NRL IPv6 - don't actually do anything */
|
|
#define SO_SECURITY_AUTHENTICATION 22
|
|
#define SO_SECURITY_ENCRYPTION_TRANSPORT 23
|
|
#define SO_SECURITY_ENCRYPTION_NETWORK 24
|
|
|
|
#define SO_BINDTODEVICE 25
|
|
|
|
/* Socket filtering */
|
|
#define SO_ATTACH_FILTER 26
|
|
#define SO_DETACH_FILTER 27
|
|
#define SO_GET_FILTER SO_ATTACH_FILTER
|
|
|
|
#define SO_PEERNAME 28
|
|
#define SO_TIMESTAMP 29
|
|
#define SCM_TIMESTAMP SO_TIMESTAMP
|
|
|
|
#define SO_ACCEPTCONN 30
|
|
|
|
#define SO_PEERSEC 31
|
|
#define SO_PASSSEC 34
|
|
#define SO_TIMESTAMPNS 35
|
|
#define SCM_TIMESTAMPNS SO_TIMESTAMPNS
|
|
|
|
#define SO_MARK 36
|
|
|
|
#define SO_TIMESTAMPING 37
|
|
#define SCM_TIMESTAMPING SO_TIMESTAMPING
|
|
|
|
#define SO_PROTOCOL 38
|
|
#define SO_DOMAIN 39
|
|
|
|
#define SO_RXQ_OVFL 40
|
|
|
|
#define SO_WIFI_STATUS 41
|
|
#define SCM_WIFI_STATUS SO_WIFI_STATUS
|
|
#define SO_PEEK_OFF 42
|
|
|
|
/* Instruct lower device to use last 4-bytes of skb data as FCS */
|
|
#define SO_NOFCS 43
|
|
|
|
#endif /* __ASM_GENERIC_SOCKET_H */
|