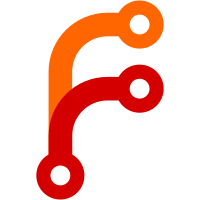
Add a wrapper routine that tells us the length of the EBDA if it is present. This guy also ensures that the returned length doesn't let the EBDA run past the 640KiB mark. Signed-off-by: Mike Waychison <mikew@google.com> Signed-off-by: Greg Kroah-Hartman <gregkh@suse.de>
57 lines
1.3 KiB
C
57 lines
1.3 KiB
C
#ifndef _ASM_X86_BIOS_EBDA_H
|
|
#define _ASM_X86_BIOS_EBDA_H
|
|
|
|
#include <asm/io.h>
|
|
|
|
/*
|
|
* there is a real-mode segmented pointer pointing to the
|
|
* 4K EBDA area at 0x40E.
|
|
*/
|
|
static inline unsigned int get_bios_ebda(void)
|
|
{
|
|
unsigned int address = *(unsigned short *)phys_to_virt(0x40E);
|
|
address <<= 4;
|
|
return address; /* 0 means none */
|
|
}
|
|
|
|
/*
|
|
* Return the sanitized length of the EBDA in bytes, if it exists.
|
|
*/
|
|
static inline unsigned int get_bios_ebda_length(void)
|
|
{
|
|
unsigned int address;
|
|
unsigned int length;
|
|
|
|
address = get_bios_ebda();
|
|
if (!address)
|
|
return 0;
|
|
|
|
/* EBDA length is byte 0 of the EBDA (stored in KiB) */
|
|
length = *(unsigned char *)phys_to_virt(address);
|
|
length <<= 10;
|
|
|
|
/* Trim the length if it extends beyond 640KiB */
|
|
length = min_t(unsigned int, (640 * 1024) - address, length);
|
|
return length;
|
|
}
|
|
|
|
void reserve_ebda_region(void);
|
|
|
|
#ifdef CONFIG_X86_CHECK_BIOS_CORRUPTION
|
|
/*
|
|
* This is obviously not a great place for this, but we want to be
|
|
* able to scatter it around anywhere in the kernel.
|
|
*/
|
|
void check_for_bios_corruption(void);
|
|
void start_periodic_check_for_corruption(void);
|
|
#else
|
|
static inline void check_for_bios_corruption(void)
|
|
{
|
|
}
|
|
|
|
static inline void start_periodic_check_for_corruption(void)
|
|
{
|
|
}
|
|
#endif
|
|
|
|
#endif /* _ASM_X86_BIOS_EBDA_H */
|