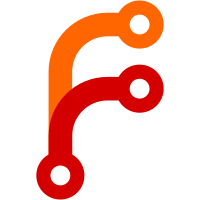
This patch makes the cpuidle_states structure global (single copy) instead of per-cpu. The statistics needed on per-cpu basis by the governor are kept per-cpu. This simplifies the cpuidle subsystem as state registration is done by single cpu only. Having single copy of cpuidle_states saves memory. Rare case of asymmetric C-states can be handled within the cpuidle driver and architectures such as POWER do not have asymmetric C-states. Having single/global registration of all the idle states, dynamic C-state transitions on x86 are handled by the boot cpu. Here, the boot cpu would disable all the devices, re-populate the states and later enable all the devices, irrespective of the cpu that would receive the notification first. Reference: https://lkml.org/lkml/2011/4/25/83 Signed-off-by: Deepthi Dharwar <deepthi@linux.vnet.ibm.com> Signed-off-by: Trinabh Gupta <g.trinabh@gmail.com> Tested-by: Jean Pihet <j-pihet@ti.com> Reviewed-by: Kevin Hilman <khilman@ti.com> Acked-by: Arjan van de Ven <arjan@linux.intel.com> Acked-by: Kevin Hilman <khilman@ti.com> Signed-off-by: Len Brown <len.brown@intel.com>
96 lines
2.1 KiB
C
96 lines
2.1 KiB
C
/*
|
|
* driver.c - driver support
|
|
*
|
|
* (C) 2006-2007 Venkatesh Pallipadi <venkatesh.pallipadi@intel.com>
|
|
* Shaohua Li <shaohua.li@intel.com>
|
|
* Adam Belay <abelay@novell.com>
|
|
*
|
|
* This code is licenced under the GPL.
|
|
*/
|
|
|
|
#include <linux/mutex.h>
|
|
#include <linux/module.h>
|
|
#include <linux/cpuidle.h>
|
|
|
|
#include "cpuidle.h"
|
|
|
|
static struct cpuidle_driver *cpuidle_curr_driver;
|
|
DEFINE_SPINLOCK(cpuidle_driver_lock);
|
|
|
|
static void __cpuidle_register_driver(struct cpuidle_driver *drv)
|
|
{
|
|
int i;
|
|
/*
|
|
* cpuidle driver should set the drv->power_specified bit
|
|
* before registering if the driver provides
|
|
* power_usage numbers.
|
|
*
|
|
* If power_specified is not set,
|
|
* we fill in power_usage with decreasing values as the
|
|
* cpuidle code has an implicit assumption that state Cn
|
|
* uses less power than C(n-1).
|
|
*
|
|
* With CONFIG_ARCH_HAS_CPU_RELAX, C0 is already assigned
|
|
* an power value of -1. So we use -2, -3, etc, for other
|
|
* c-states.
|
|
*/
|
|
if (!drv->power_specified) {
|
|
for (i = CPUIDLE_DRIVER_STATE_START; i < drv->state_count; i++)
|
|
drv->states[i].power_usage = -1 - i;
|
|
}
|
|
}
|
|
|
|
|
|
/**
|
|
* cpuidle_register_driver - registers a driver
|
|
* @drv: the driver
|
|
*/
|
|
int cpuidle_register_driver(struct cpuidle_driver *drv)
|
|
{
|
|
if (!drv)
|
|
return -EINVAL;
|
|
|
|
if (cpuidle_disabled())
|
|
return -ENODEV;
|
|
|
|
spin_lock(&cpuidle_driver_lock);
|
|
if (cpuidle_curr_driver) {
|
|
spin_unlock(&cpuidle_driver_lock);
|
|
return -EBUSY;
|
|
}
|
|
__cpuidle_register_driver(drv);
|
|
cpuidle_curr_driver = drv;
|
|
spin_unlock(&cpuidle_driver_lock);
|
|
|
|
return 0;
|
|
}
|
|
|
|
EXPORT_SYMBOL_GPL(cpuidle_register_driver);
|
|
|
|
/**
|
|
* cpuidle_get_driver - return the current driver
|
|
*/
|
|
struct cpuidle_driver *cpuidle_get_driver(void)
|
|
{
|
|
return cpuidle_curr_driver;
|
|
}
|
|
EXPORT_SYMBOL_GPL(cpuidle_get_driver);
|
|
|
|
/**
|
|
* cpuidle_unregister_driver - unregisters a driver
|
|
* @drv: the driver
|
|
*/
|
|
void cpuidle_unregister_driver(struct cpuidle_driver *drv)
|
|
{
|
|
if (drv != cpuidle_curr_driver) {
|
|
WARN(1, "invalid cpuidle_unregister_driver(%s)\n",
|
|
drv->name);
|
|
return;
|
|
}
|
|
|
|
spin_lock(&cpuidle_driver_lock);
|
|
cpuidle_curr_driver = NULL;
|
|
spin_unlock(&cpuidle_driver_lock);
|
|
}
|
|
|
|
EXPORT_SYMBOL_GPL(cpuidle_unregister_driver);
|