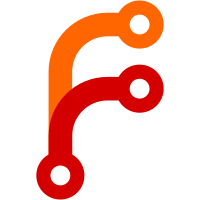
Add support for a legacy mapping where irq = (hwirq - first_hwirq + first_irq) so that a controller driver can allocate a fixed range of irq_descs and use a simple calculation to translate back and forth between linux and hw irq numbers. This is needed to use an irq_domain with many of the ARM interrupt controller drivers that manage their own irq_desc allocations. Ultimately the goal is to migrate those drivers to use the linear revmap, but doing it this way allows each driver to be converted separately which makes the migration path easier. This patch generalizes the IRQ_DOMAIN_MAP_LEGACY method to use (first_irq-first_hwirq) as the offset between hwirq and linux irq number, and adds checks to make sure that the hwirq number does not exceed range assigned to the controller. Signed-off-by: Grant Likely <grant.likely@secretlab.ca> Cc: Rob Herring <rob.herring@calxeda.com> Cc: Benjamin Herrenschmidt <benh@kernel.crashing.org> Cc: Thomas Gleixner <tglx@linutronix.de> Cc: Milton Miller <miltonm@bga.com> Tested-by: Olof Johansson <olof@lixom.net>
91 lines
2.3 KiB
C
91 lines
2.3 KiB
C
#ifdef __KERNEL__
|
|
#ifndef _ASM_POWERPC_IRQ_H
|
|
#define _ASM_POWERPC_IRQ_H
|
|
|
|
/*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version
|
|
* 2 of the License, or (at your option) any later version.
|
|
*/
|
|
|
|
#include <linux/irqdomain.h>
|
|
#include <linux/threads.h>
|
|
#include <linux/list.h>
|
|
#include <linux/radix-tree.h>
|
|
|
|
#include <asm/types.h>
|
|
#include <linux/atomic.h>
|
|
|
|
|
|
/* Define a way to iterate across irqs. */
|
|
#define for_each_irq(i) \
|
|
for ((i) = 0; (i) < NR_IRQS; ++(i))
|
|
|
|
extern atomic_t ppc_n_lost_interrupts;
|
|
|
|
/* This number is used when no interrupt has been assigned */
|
|
#define NO_IRQ (0)
|
|
|
|
/* This is a special irq number to return from get_irq() to tell that
|
|
* no interrupt happened _and_ ignore it (don't count it as bad). Some
|
|
* platforms like iSeries rely on that.
|
|
*/
|
|
#define NO_IRQ_IGNORE ((unsigned int)-1)
|
|
|
|
/* Total number of virq in the platform */
|
|
#define NR_IRQS CONFIG_NR_IRQS
|
|
|
|
/* Same thing, used by the generic IRQ code */
|
|
#define NR_IRQS_LEGACY NUM_ISA_INTERRUPTS
|
|
|
|
struct irq_data;
|
|
extern irq_hw_number_t irqd_to_hwirq(struct irq_data *d);
|
|
extern irq_hw_number_t virq_to_hw(unsigned int virq);
|
|
|
|
/**
|
|
* irq_early_init - Init irq remapping subsystem
|
|
*/
|
|
extern void irq_early_init(void);
|
|
|
|
static __inline__ int irq_canonicalize(int irq)
|
|
{
|
|
return irq;
|
|
}
|
|
|
|
extern int distribute_irqs;
|
|
|
|
struct irqaction;
|
|
struct pt_regs;
|
|
|
|
#define __ARCH_HAS_DO_SOFTIRQ
|
|
|
|
#if defined(CONFIG_BOOKE) || defined(CONFIG_40x)
|
|
/*
|
|
* Per-cpu stacks for handling critical, debug and machine check
|
|
* level interrupts.
|
|
*/
|
|
extern struct thread_info *critirq_ctx[NR_CPUS];
|
|
extern struct thread_info *dbgirq_ctx[NR_CPUS];
|
|
extern struct thread_info *mcheckirq_ctx[NR_CPUS];
|
|
extern void exc_lvl_ctx_init(void);
|
|
#else
|
|
#define exc_lvl_ctx_init()
|
|
#endif
|
|
|
|
/*
|
|
* Per-cpu stacks for handling hard and soft interrupts.
|
|
*/
|
|
extern struct thread_info *hardirq_ctx[NR_CPUS];
|
|
extern struct thread_info *softirq_ctx[NR_CPUS];
|
|
|
|
extern void irq_ctx_init(void);
|
|
extern void call_do_softirq(struct thread_info *tp);
|
|
extern int call_handle_irq(int irq, void *p1,
|
|
struct thread_info *tp, void *func);
|
|
extern void do_IRQ(struct pt_regs *regs);
|
|
|
|
int irq_choose_cpu(const struct cpumask *mask);
|
|
|
|
#endif /* _ASM_IRQ_H */
|
|
#endif /* __KERNEL__ */
|