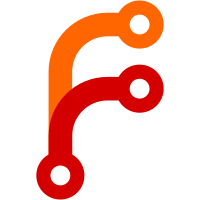
The AT91CAP9 revC CPU has a few differences over the previous, revB CPU which was distributed in small quantities only (revA was an internal Atmel product only). This patch adds the detection routines to recognize the different AT91CAP9 revisions (based on the PMC subsystem version number), and uses them to: - activate a workaround for the external interrupts levels (on revB CPUs) - set the UDPHS_BYPASS bit (on revB CPUs) - set AT91_GPBR register address to the correct offset (0xfffffd50 on revB, 0xfffffd60 on revC) For debugging usage, the CPU revision can be found in /proc/cpuinfo on the 'Revision' line. This patch is extracted from Andrew Victor's -at91 patch (2.6.27-at91.patch) where it has been tested for the last 6 months. Signed-off-by: Stelian Pop <stelian@popies.net> Signed-off-by: Andrew Victor <linux@maxim.org.za> Signed-off-by: Russell King <rmk+kernel@arm.linux.org.uk>
118 lines
3.1 KiB
C
118 lines
3.1 KiB
C
/*
|
|
* arch/arm/mach-at91/include/mach/cpu.h
|
|
*
|
|
* Copyright (C) 2006 SAN People
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
*/
|
|
|
|
#ifndef __ASM_ARCH_CPU_H
|
|
#define __ASM_ARCH_CPU_H
|
|
|
|
#include <mach/hardware.h>
|
|
#include <mach/at91_dbgu.h>
|
|
|
|
|
|
#define ARCH_ID_AT91RM9200 0x09290780
|
|
#define ARCH_ID_AT91SAM9260 0x019803a0
|
|
#define ARCH_ID_AT91SAM9261 0x019703a0
|
|
#define ARCH_ID_AT91SAM9263 0x019607a0
|
|
#define ARCH_ID_AT91SAM9G20 0x019905a0
|
|
#define ARCH_ID_AT91SAM9RL64 0x019b03a0
|
|
#define ARCH_ID_AT91CAP9 0x039A03A0
|
|
|
|
#define ARCH_ID_AT91SAM9XE128 0x329973a0
|
|
#define ARCH_ID_AT91SAM9XE256 0x329a93a0
|
|
#define ARCH_ID_AT91SAM9XE512 0x329aa3a0
|
|
|
|
#define ARCH_ID_AT91M40800 0x14080044
|
|
#define ARCH_ID_AT91R40807 0x44080746
|
|
#define ARCH_ID_AT91M40807 0x14080745
|
|
#define ARCH_ID_AT91R40008 0x44000840
|
|
|
|
static inline unsigned long at91_cpu_identify(void)
|
|
{
|
|
return (at91_sys_read(AT91_DBGU_CIDR) & ~AT91_CIDR_VERSION);
|
|
}
|
|
|
|
|
|
#define ARCH_FAMILY_AT91X92 0x09200000
|
|
#define ARCH_FAMILY_AT91SAM9 0x01900000
|
|
#define ARCH_FAMILY_AT91SAM9XE 0x02900000
|
|
|
|
static inline unsigned long at91_arch_identify(void)
|
|
{
|
|
return (at91_sys_read(AT91_DBGU_CIDR) & AT91_CIDR_ARCH);
|
|
}
|
|
|
|
#ifdef CONFIG_ARCH_AT91CAP9
|
|
#include <mach/at91_pmc.h>
|
|
|
|
#define ARCH_REVISION_CAP9_B 0x399
|
|
#define ARCH_REVISION_CAP9_C 0x601
|
|
|
|
static inline unsigned long at91cap9_rev_identify(void)
|
|
{
|
|
return (at91_sys_read(AT91_PMC_VER));
|
|
}
|
|
#endif
|
|
|
|
#ifdef CONFIG_ARCH_AT91RM9200
|
|
#define cpu_is_at91rm9200() (at91_cpu_identify() == ARCH_ID_AT91RM9200)
|
|
#else
|
|
#define cpu_is_at91rm9200() (0)
|
|
#endif
|
|
|
|
#ifdef CONFIG_ARCH_AT91SAM9260
|
|
#define cpu_is_at91sam9xe() (at91_arch_identify() == ARCH_FAMILY_AT91SAM9XE)
|
|
#define cpu_is_at91sam9260() ((at91_cpu_identify() == ARCH_ID_AT91SAM9260) || cpu_is_at91sam9xe())
|
|
#else
|
|
#define cpu_is_at91sam9xe() (0)
|
|
#define cpu_is_at91sam9260() (0)
|
|
#endif
|
|
|
|
#ifdef CONFIG_ARCH_AT91SAM9G20
|
|
#define cpu_is_at91sam9g20() (at91_cpu_identify() == ARCH_ID_AT91SAM9G20)
|
|
#else
|
|
#define cpu_is_at91sam9g20() (0)
|
|
#endif
|
|
|
|
#ifdef CONFIG_ARCH_AT91SAM9261
|
|
#define cpu_is_at91sam9261() (at91_cpu_identify() == ARCH_ID_AT91SAM9261)
|
|
#else
|
|
#define cpu_is_at91sam9261() (0)
|
|
#endif
|
|
|
|
#ifdef CONFIG_ARCH_AT91SAM9263
|
|
#define cpu_is_at91sam9263() (at91_cpu_identify() == ARCH_ID_AT91SAM9263)
|
|
#else
|
|
#define cpu_is_at91sam9263() (0)
|
|
#endif
|
|
|
|
#ifdef CONFIG_ARCH_AT91SAM9RL
|
|
#define cpu_is_at91sam9rl() (at91_cpu_identify() == ARCH_ID_AT91SAM9RL64)
|
|
#else
|
|
#define cpu_is_at91sam9rl() (0)
|
|
#endif
|
|
|
|
#ifdef CONFIG_ARCH_AT91CAP9
|
|
#define cpu_is_at91cap9() (at91_cpu_identify() == ARCH_ID_AT91CAP9)
|
|
#define cpu_is_at91cap9_revB() (at91cap9_rev_identify() == ARCH_REVISION_CAP9_B)
|
|
#define cpu_is_at91cap9_revC() (at91cap9_rev_identify() == ARCH_REVISION_CAP9_C)
|
|
#else
|
|
#define cpu_is_at91cap9() (0)
|
|
#define cpu_is_at91cap9_revB() (0)
|
|
#define cpu_is_at91cap9_revC() (0)
|
|
#endif
|
|
|
|
/*
|
|
* Since this is ARM, we will never run on any AVR32 CPU. But these
|
|
* definitions may reduce clutter in common drivers.
|
|
*/
|
|
#define cpu_is_at32ap7000() (0)
|
|
|
|
#endif
|