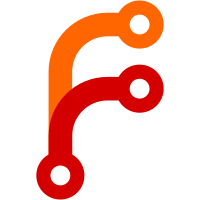
Initial git repository build. I'm not bothering with the full history, even though we have it. We can create a separate "historical" git archive of that later if we want to, and in the meantime it's about 3.2GB when imported into git - space that would just make the early git days unnecessarily complicated, when we don't have a lot of good infrastructure for it. Let it rip!
40 lines
963 B
C
40 lines
963 B
C
#include <linux/module.h>
|
|
#include <linux/spinlock.h>
|
|
#include <asm/atomic.h>
|
|
|
|
/*
|
|
* This is an architecture-neutral, but slow,
|
|
* implementation of the notion of "decrement
|
|
* a reference count, and return locked if it
|
|
* decremented to zero".
|
|
*
|
|
* NOTE NOTE NOTE! This is _not_ equivalent to
|
|
*
|
|
* if (atomic_dec_and_test(&atomic)) {
|
|
* spin_lock(&lock);
|
|
* return 1;
|
|
* }
|
|
* return 0;
|
|
*
|
|
* because the spin-lock and the decrement must be
|
|
* "atomic".
|
|
*
|
|
* This slow version gets the spinlock unconditionally,
|
|
* and releases it if it isn't needed. Architectures
|
|
* are encouraged to come up with better approaches,
|
|
* this is trivially done efficiently using a load-locked
|
|
* store-conditional approach, for example.
|
|
*/
|
|
|
|
#ifndef ATOMIC_DEC_AND_LOCK
|
|
int _atomic_dec_and_lock(atomic_t *atomic, spinlock_t *lock)
|
|
{
|
|
spin_lock(lock);
|
|
if (atomic_dec_and_test(atomic))
|
|
return 1;
|
|
spin_unlock(lock);
|
|
return 0;
|
|
}
|
|
|
|
EXPORT_SYMBOL(_atomic_dec_and_lock);
|
|
#endif
|