prepare stuff for public code dump
parent
a1740ebbec
commit
61dbe86c2b
|
@ -0,0 +1,14 @@
|
|||
DO WHAT THE FUCK YOU WANT TO PUBLIC LICENSE
|
||||
Version 2, December 2004
|
||||
|
||||
Copyright (C) 2004 Sam Hocevar <sam@hocevar.net>
|
||||
|
||||
Everyone is permitted to copy and distribute verbatim or modified
|
||||
copies of this license document, and changing it is allowed as long
|
||||
as the name is changed.
|
||||
|
||||
DO WHAT THE FUCK YOU WANT TO PUBLIC LICENSE
|
||||
TERMS AND CONDITIONS FOR COPYING, DISTRIBUTION AND MODIFICATION
|
||||
|
||||
0. You just DO WHAT THE FUCK YOU WANT TO.
|
||||
|
|
@ -0,0 +1,54 @@
|
|||
bigbrain
|
||||
===
|
||||
|
||||
An experiment in following
|
||||
[neuralnetworksanddeeplearning.com](http://neuralnetworksanddeeplearning.com/)
|
||||
in Rust. The end result is a web page that can sometimes recognize hand-written
|
||||
digits using a three-layer (one hidden) neural network.
|
||||
|
||||
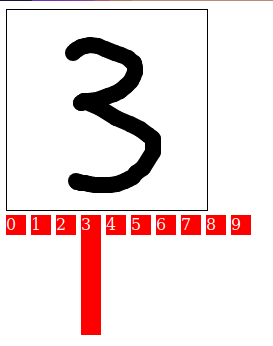
|
||||
|
||||
You can see it in action at [https://hackerspace.pl/~q3k/bigbrain/main.html](https://hackerspace.pl/~q3k/bigbrain/main.html). It's really good at figuring out the digit 2, and gets somewhat confused about other digits. Be kind to it.
|
||||
|
||||
Goals
|
||||
---
|
||||
|
||||
1. Be a weekend project
|
||||
2. Implement everything specific to ML/DL/NN from scratch
|
||||
3. End up with a janky JS/WASM demo
|
||||
|
||||
Non-Goals
|
||||
---
|
||||
|
||||
1. Be fast
|
||||
2. Be good
|
||||
3. Be clean code
|
||||
|
||||
Training
|
||||
---
|
||||
|
||||
First, acquire the MNIST handwritten digit database (training/test sets, both images and labels) and save them in this repo.
|
||||
|
||||
Then, `cargo run --release` to run training, which will generate a `net.pb` containing the trained model.
|
||||
|
||||
Building web app
|
||||
---
|
||||
|
||||
You'll need wasm-pack (`cargo install wasm-pack`). Then:
|
||||
|
||||
```
|
||||
cd bigbrainjs
|
||||
wasm-pack build --release --target web
|
||||
```
|
||||
|
||||
You can then serve files from the `bigbrainjs/web` repository to see the web interface. The simplest way to do that is probably to run `python -m http.server`.
|
||||
|
||||
|
||||
License
|
||||
===
|
||||
|
||||
Copyright © 2021-2023 Serge Bazanski <q3k@q3k.org>
|
||||
|
||||
This work is free. You can redistribute it and/or modify it under the
|
||||
terms of the Do What The Fuck You Want To Public License, Version 2,
|
||||
as published by Sam Hocevar. See the COPYING file for more details.
|
29
src/main.rs
29
src/main.rs
|
@ -5,8 +5,9 @@ use bigbrain::{mnist,network,proto::network as npb};
|
|||
use protobuf::Message;
|
||||
|
||||
fn main() {
|
||||
env_logger::init();
|
||||
env_logger::init_from_env( env_logger::Env::default().filter_or(env_logger::DEFAULT_FILTER_ENV, "info"));
|
||||
|
||||
log::info!("Loading training/test images...");
|
||||
let now = time::Instant::now();
|
||||
let train = mnist::load("train").unwrap();
|
||||
let test = mnist::load("t10k").unwrap();
|
||||
|
@ -18,19 +19,19 @@ fn main() {
|
|||
log::info!("Split into {} training and {} validation", num_train, num_validation);
|
||||
let (train, validation) = train.split(num_train);
|
||||
|
||||
//let now = time::Instant::now();
|
||||
//let size = vec![
|
||||
// 28*28 as usize,
|
||||
// 30usize,
|
||||
// 10usize,
|
||||
//];
|
||||
//let mut net = network::Network::new(&size);
|
||||
//let elapsed = now.elapsed();
|
||||
//log::info!("Created random network {:?} in {:?}.", size, elapsed);
|
||||
//net.sgd(&train, 30, 32, 3.0, Some(&test));
|
||||
//let proto = net.proto();
|
||||
//let mut f = std::fs::File::create("net.pb").unwrap();
|
||||
//proto.write_to_writer(&mut f).unwrap();
|
||||
let now = time::Instant::now();
|
||||
let size = vec![
|
||||
28*28 as usize,
|
||||
30usize,
|
||||
10usize,
|
||||
];
|
||||
let mut net = network::Network::new(&size);
|
||||
let elapsed = now.elapsed();
|
||||
log::info!("Created random network {:?} in {:?}.", size, elapsed);
|
||||
net.sgd(&train, 30, 32, 3.0, Some(&test));
|
||||
let proto = net.proto();
|
||||
let mut f = std::fs::File::create("net.pb").unwrap();
|
||||
proto.write_to_writer(&mut f).unwrap();
|
||||
|
||||
let nbytes = std::fs::read("net.pb").unwrap();
|
||||
let n = npb::Network::parse_from_bytes(&nbytes).unwrap();
|
||||
|
|
Loading…
Reference in New Issue